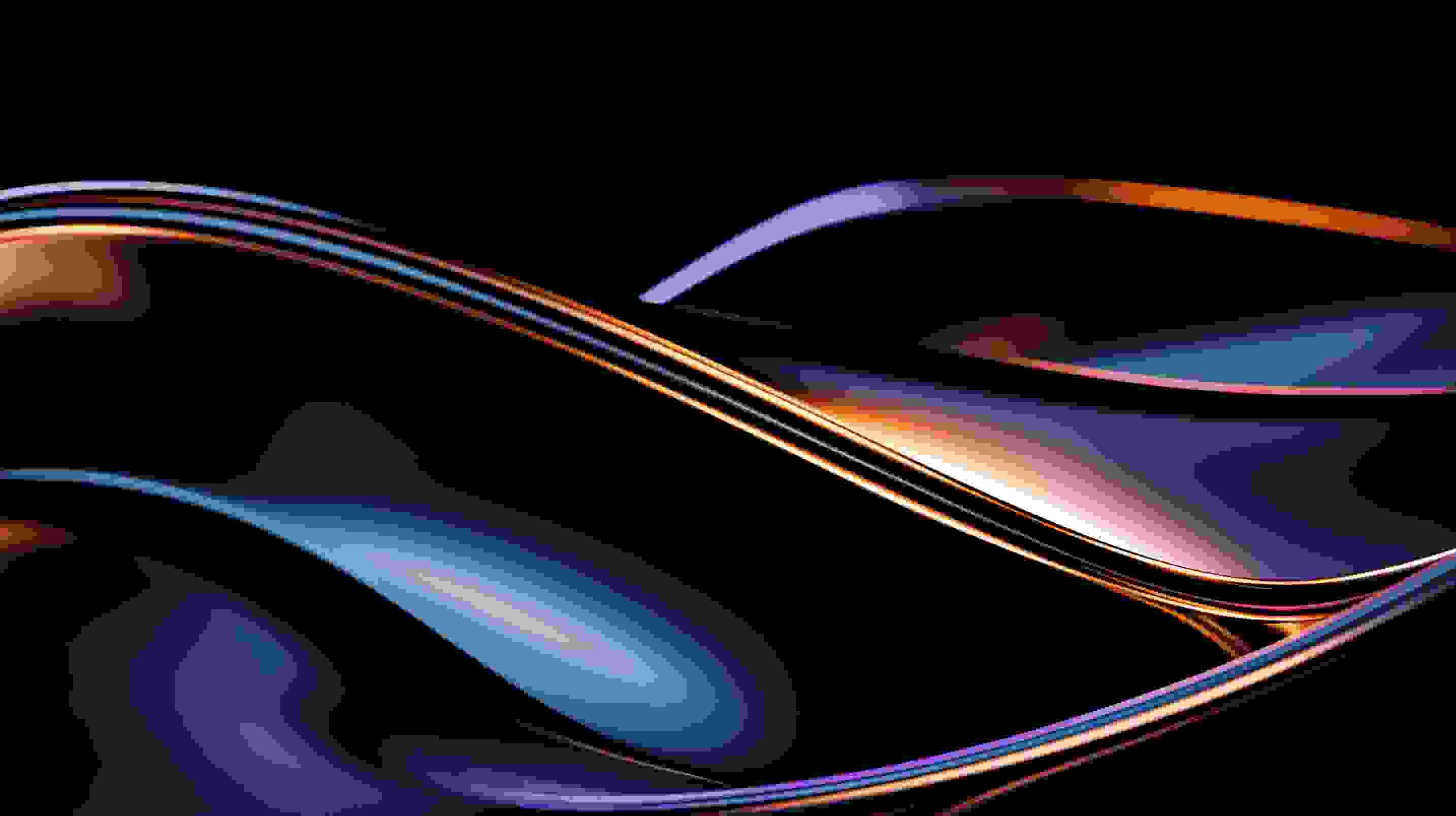
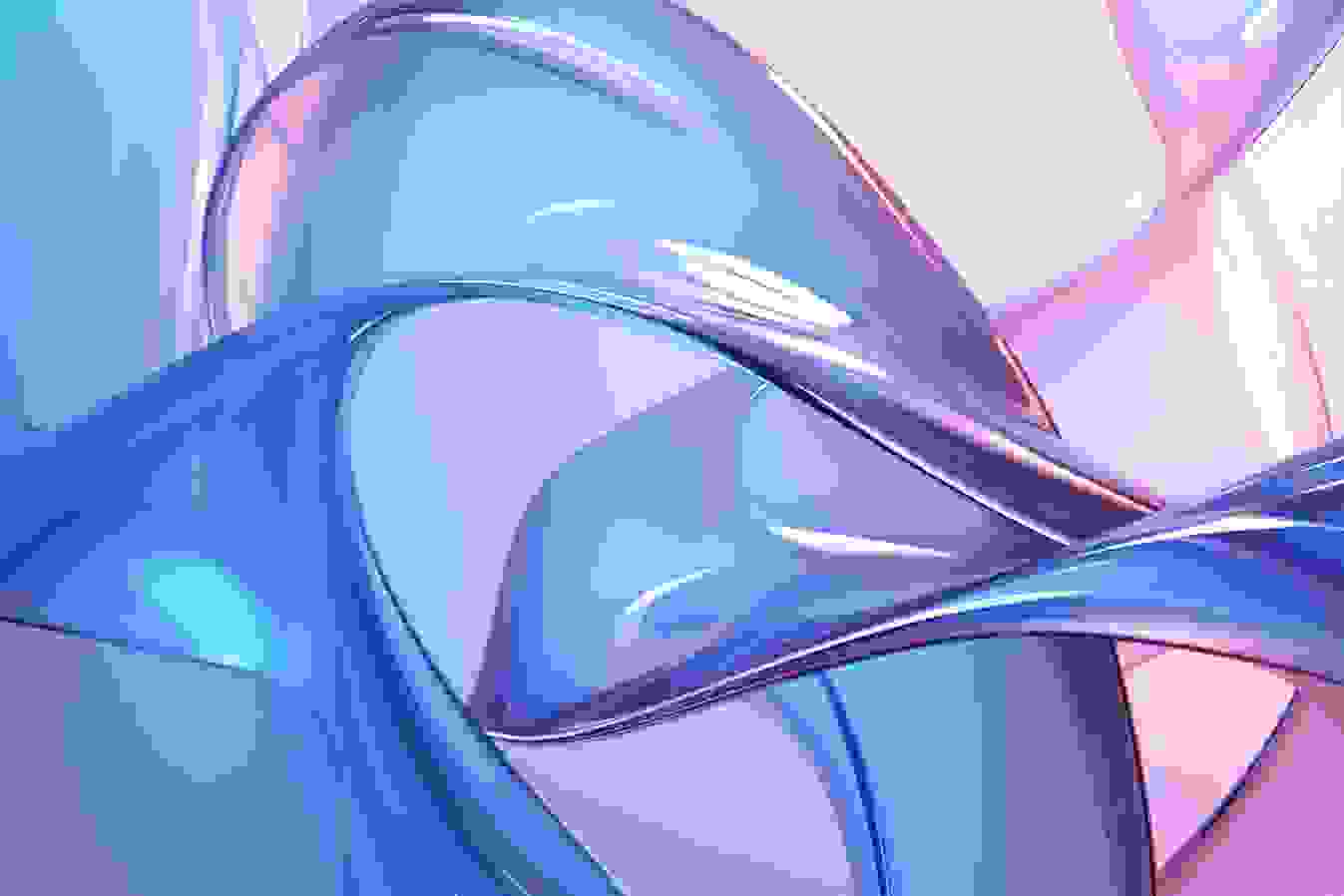
Front End
1 minutes read
Discussing about a global state management choice, redux would mostly become the chosen package in few years ago. But, in the last few years there's come many options to handle a global state management. Among others, one i think that stands out is Zustand, a state management package that was first released on 2020. The one things that make me think this package is stand out is its simplicity and effectiveness at handling state management, it also has the smallest bundle size.
The term simple and effective that i use here is referring to how quick we can create and use a state management store using Zustand. To create a store, we also only need to directly define the state value and the dispacther functions within its create store functions. Bellow is a simple example on how a Zustand store created.
1import { create } from 'zustand';
2
3const useCountStore = create((set) => ({
4 count: 0,
5 increment: (qty) => set((state) => ({ count: state.count + qty })),
6 decrement: (qty) => set((state) => ({ count: state.count - qty })),
7}))
To use those store value and dispacth function, we also only need to call the created Zustand custom hook store function within the component that need it.
1const Component = () => {
2 const count = useCountStore((state) => state.count)
3 const increment = useCountStore((state) => state.increment)
4 const decrement = useCountStore((state) => state.decrement)
5 // ...
6}
Furthermore, to simplify how we use the store, we could define a selectors for every item in the store, so we wouldn't to write a function to target the store items everytime we use it. To define the selectors, we would only need one extra lines of code.
1import { create, createSelectors } from 'zustand';
2
3const countStore = create((set) => ({
4 count: 0,
5 increment: (qty) => set((state) => ({ count: state.count + qty })),
6 decrement: (qty) => set((state) => ({ count: state.count - qty })),
7}))
8
9const useCountStore = createSelectors(countStore)
10
11const Component = () => {
12 const count = useCountStore.use.count()
13 const increment = useCountStore.use.increment()
14 // ...
15}
After reading all above explanation, you would also notice another things that made this package stands out. It's the easiness to learn and understand how it works.